Script-2-API Usage
Script-2-API allows you to create a REST API by creating endpoints with JavaScript or Groovy script languages without the need for any code editor.
With specially defined variables, it is possible to use, update or delete message sections in the code.
In this content, Script-2-API definition for a REST API where mail operations are managed, creating a sendMail endpoint for sending mail, creating and installing and testing REST API as API Proxy will be explained.
1. Creating Script-2-API
REST API definition is made by entering general information of the API easily on the Script-2-API screen.
1.1.Creating the /sendMail Enpoint
In the image below, the /sendMail endpoint generates the email sending process in Groovy language by taking the email information from the body of the request message.
To add an endpoint, click the Add button, enter the endpoint information, and click the Save button.
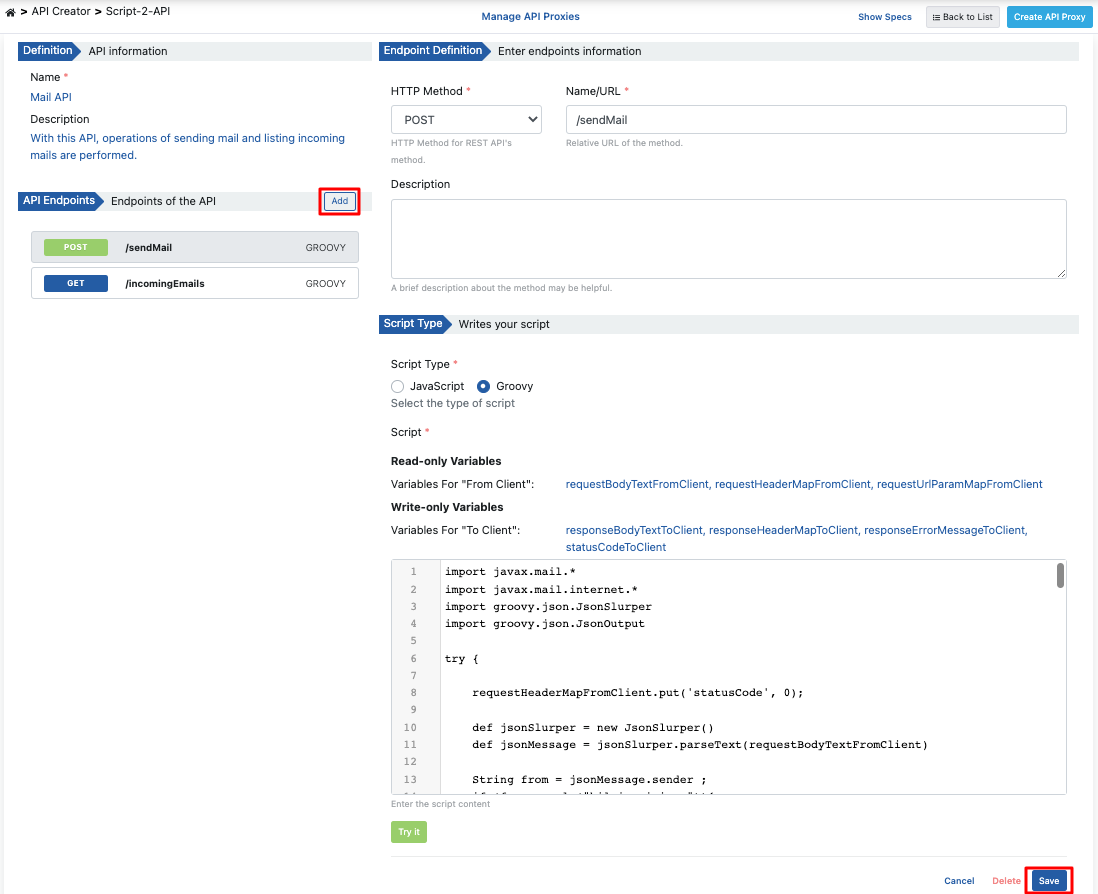
The script code can be tested by clicking the Try It button.
Groovy code;
import javax.mail.*
import javax.mail.internet.*
import groovy.json.JsonSlurper
import groovy.json.JsonOutput
try {
requestHeaderMapFromClient.put('statusCode', 0);
def jsonSlurper = new JsonSlurper()
def jsonMessage = jsonSlurper.parseText(requestBodyTextFromClient)
String from = jsonMessage.sender ;
if (from.equals("bilgi.apinizer")){
from="info@apinizer.com";
} else if(from.equals("apinizer.hata")){
from="warning@apinizer.com";
} else {
statusCode=400;
requestErrorMessageFromClient = JsonOutput.toJson([isTheOperationSuccessful: false, errorMessage: 'Hatalı gönderen bilgisi', errorCode:'01-ERR-02'])
return;
}
String to=jsonMessage.reciever;
String cc=jsonMessage.recieverInformation;
String bcc=jsonMessage.recieverInformation;
if(!to?.trim() && !cc?.trim() && !bcc?.trim()){
requestErrorMessageFromClient = JsonOutput.toJson([isTheOperationSuccessful: false, errorMessage: 'En az bir alıcı eklenmeli', errorCode:'01-ERR-03'])
statusCode=400;
return;
}
String subject=jsonMessage.subject;
if(subject?.trim()){
subject=""
}
String content=jsonMessage.content;
if(content?.trim()){
content=""
}
String mimeType=jsonMessage.contentType;
if(mimeType?.trim()){
mimeType="text/html;charset=utf-8"
}
// Init constants of sender email account.
String email = "apinizer.hata"
String password = ""
String host = "mail.apinizer.com"
String port = "25" // "465" "587"
// Set up properties.
Properties props = System.getProperties()
props.put("mail.smtp.user", email)
props.put("mail.smtp.host", host)
props.put("mail.smtp.port", port)
props.put("mail.smtp.auth", "false")
props.put("mail.smtp.starttls.enable","false")
props.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory")
props.put("mail.smtp.ssl.trust", host) // Change host to "*" if you want to trust all host.
Session session = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(email, password);
}
});
// Set up message.
MimeMessage message = new MimeMessage(session)
message.setFrom(new InternetAddress(from))
if(to?.trim()){
message.addRecipients(Message.RecipientType.TO, new InternetAddress(to))
}
if(cc?.trim()){
message.addRecipients(Message.RecipientType.CC, new InternetAddress(cc))
}
if(bcc?.trim()){
message.addRecipients(Message.RecipientType.BCC, new InternetAddress(bcc))
}
message.setSubject(subject)
message.setContent(content, mimeType)
try {
// Send mail.
Transport.send(message )
} catch (MessagingException e) {
e.printStackTrace();
statusCode=500;
responseErrorMessageToClient = JsonOutput.toJson([isTheOperationSuccessful: false, errorMessage: 'Error occurred while sending mail:' + e.getMessage(), errorCode:'01-ERR-04'])
return;
}
statusCode=200;
responseBodyTextToClient = JsonOutput.toJson([isTheOperationSuccessful: true, errorMessage: '', errorCode:''])
} catch (MessagingException e) {
e.printStackTrace();
statusCode=500;
responseErrorMessageToClient = JsonOutput.toJson([isTheOperationSuccessful: false, errorMessage: 'Error occurred while sending mail:' + e.getMessage(), errorCode:'01-ERR-05'])
return;
}
When sending email, the body of the request must be the lower JSON body;
{
"sender":"info@apinizer.com"",
"reciever":"user@apinizer.com",
"recieverInformation":null,
"secretReceivers":null,
"subject":"test",
"content":"test",
"contentType":"text/html;charset=utf-8"
}
When a successful response is returned, the message template example below is encountered;
{
"isTheOperationSuccessful":true,
"errorMessage":"",
"errorCode":""
}
When an incorrect response is returned, the message template example in the below template is encountered;
Script Error: {
"isTheOperationSuccessful":false,
"errorMessage":"Error occurred while sending mail:Invalid Addresses",
"errorCode":"01-ERR-04"
}
2. Viewing API Definition Documents
Click the Show Specs link to access the API definition files of the Mail API.
The picture below shows the Spec Information dialog:
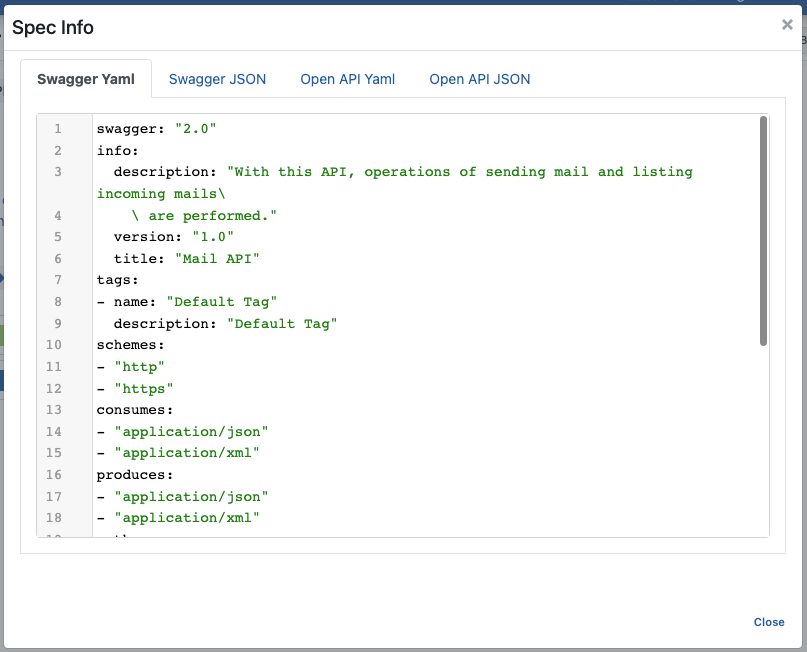
3. Creating API Proxy
API Proxy can be created instantly by clicking the Create API Proxy button on the Script-2-API screen.
While creating the API Proxy, fill in the input fields in the image below and click the Save button.
3.1 Go Live API Proxy
Mail API Proxy can be quickly deployed on the defined environment and opened for consumption by clients.
The management of this process can be done through the dialog opened with the Deploy button on the API Proxy screen.
This process can also be managed from the Manage API Proxies link on the Script-2-API screen.

4. Testing
Below is the image of testing the /sendMail endpoint on the API Proxy page;